Devlog #4: Creating Puzzle Slots
Adding Children to C++ UUserWidget
Anyhow, in a past devlog, I talked about adding widgets through C++ using BindWidget. However, this time, I wanted to not only create widget components, but also to add them to my class in a hierarchy (ie; having a UImage be a child of a USizebox). I found useful information on this forum post:
I wrote the following code in my NativeConstruct override:
NOTE FROM FUTURE ABYSS: WHILE THIS METHOD DOES INDEED WORK, IT CAUSED ISSUES FOR ME AND WOULDN'T APPEAR VISIBLE AT RUN TIME NO MATTER WHAT. I ENDED UP COMMENTING IT OUT IN THE END.
void UJigsawSlot::NativeConstruct() { SizeBox = WidgetTree->ConstructWidget<USizeBox>(USizeBox::StaticClass(), TEXT("RootWidget")); WidgetTree->RootWidget = SizeBox; SizeBox->AddChild(Image); }
And this method did indeed work; when I created the appropriate widgets in the editor, it wouldn't let me add an Image widget component that is a child of the root, it only let me add an image component that is a child of the sizebox:
Creating Event Dispatchers in C++
Afterwards, I needed to add event dispatchers through C++ in a way that they could be run by blueprints. I've done event dispatchers before, but it's been a while so I needed a refresher.
I used the instructions from this forum post:
C++ Event dispatchers: How do we implement them?
And this here is the code I wrote:
JigsawSlot.h
// Fill out your copyright notice in the Description page of Project Settings. #pragma once #include "CoreMinimal.h" #include "Blueprint/UserWidget.h" #include "JigsawSlot.generated.h" //delegates must be created outside fo the class declaration //delegate type declarations must start with F DECLARE_DYNAMIC_MULTICAST_DELEGATE(FPieceCorrectlyPlacedDelegate); // We make the class abstract, as we don't want to create // instances of this, instead we want to create instances // of our UMG Blueprint subclass. UCLASS(Abstract) class PUZZLEGAME_API UJigsawSlot : public UUserWidget { GENERATED_BODY() public: /* Widgets*/ UPROPERTY(BlueprintReadWrite, Category = "Puzzle", meta = (BindWidget)) class USizeBox* SizeBox; UPROPERTY(BlueprintReadWrite, Category = "Puzzle", meta = (BindWidget)) class UImage* Image; /* Variables*/ UPROPERTY(BlueprintReadWrite, Category = "Puzzle", meta = (ExposeOnSpawn = "true")) FVector2D SlotCoordinate = FVector2D(0, 0); UPROPERTY(BlueprintReadWrite, Category = "Puzzle", meta = (ExposeOnSpawn = "true")) UTexture* JigsawImage; UPROPERTY(BlueprintReadWrite, Category = "Puzzle", meta = (ExposeOnSpawn = "true")) int NumberOfPieces; /* Delegates/ Event Dispatchers*/ UPROPERTY(BlueprintAssignable, Category = "Delegate") FPieceCorrectlyPlacedDelegate OnPieceCorrectlyPlaced; protected: // Doing setup in the C++ constructor is not as useful as using NativeConstruct. virtual void NativeConstruct() override; };
JigsawSlot.cpp
// Fill out your copyright notice in the Description page of Project Settings. #include "JigsawSlot.h" #include "Components/Image.h" #include "Components/SizeBox.h" #include "Blueprint/WidgetTree.h" void UJigsawSlot::NativeConstruct() { SizeBox = WidgetTree->ConstructWidget<USizeBox>(USizeBox::StaticClass(), TEXT("RootWidget")); WidgetTree->RootWidget = SizeBox; SizeBox->AddChild(Image); }
And this code worked great! I was able to implement the Event Dispatcher in blueprints:
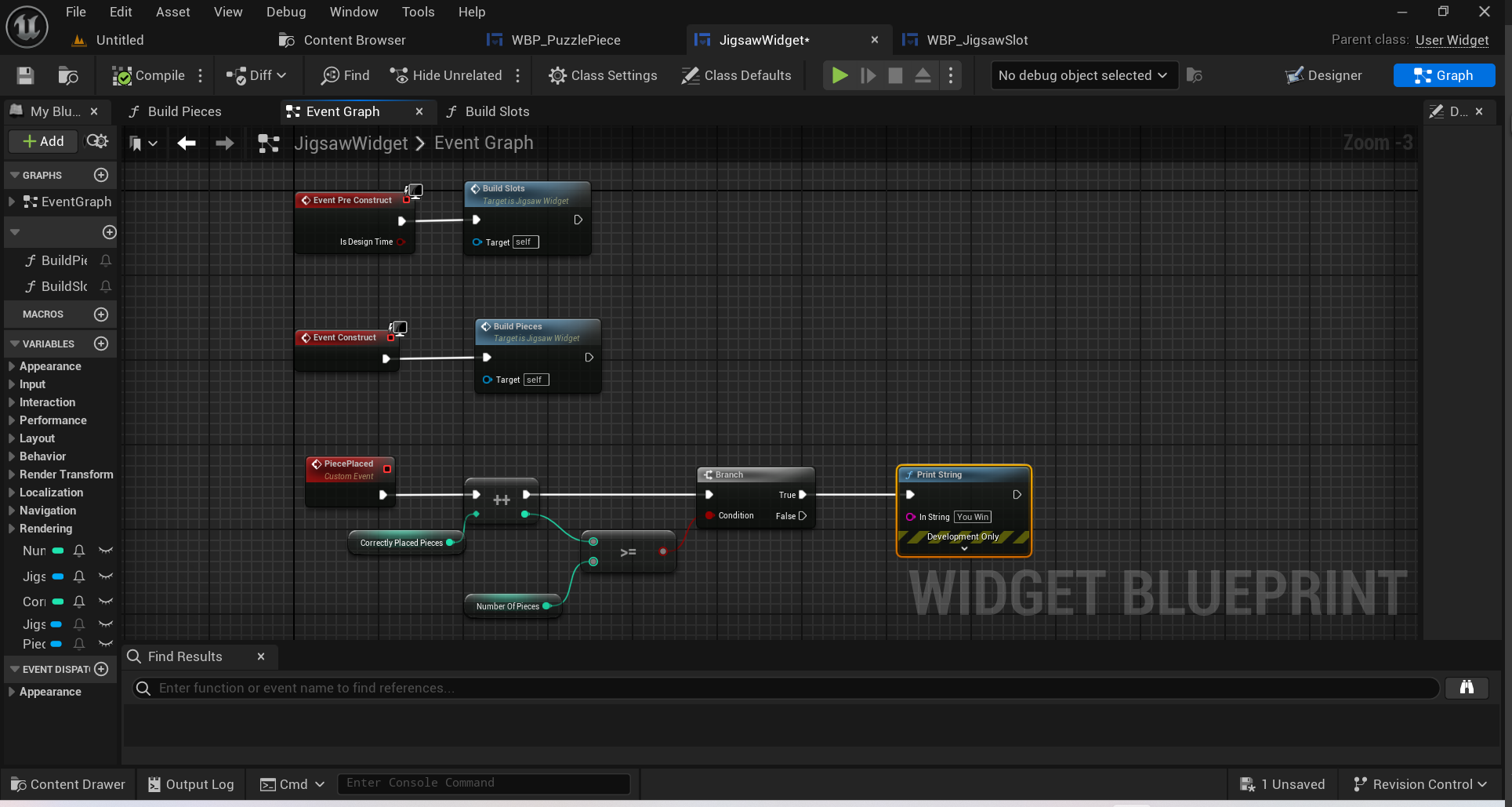
Get Fyolai Puzzle Game
Fyolai Puzzle Game
A simple puzzle game for a school project. Wouldn't recommend playing it 💀
Status | Released |
Author | AbyssEclipse |
Genre | Puzzle |
More posts
- Jigsaw Puzzle- Post Mortem53 days ago
- Devlog 5: Drag and Drop53 days ago
- Devlog #3: Creating Puzzle Piece Tray53 days ago
- Devlog 2: Creating Puzzle Piece in C++54 days ago
- Devlog 1: Puzzle Material55 days ago
Leave a comment
Log in with itch.io to leave a comment.